UI Hint Methods
The table below lists the UI hint methods that are recognized as part of default programming model.
Method | Description |
---|---|
Provides a CSS class for this object instance.
In conjunction with |
|
Provides the name of the image to render, usually alongside the title, to represent the object. If not provided, then the class name is used to locate an image. |
|
Provides an alternative layout to use, if available. If null or no such layout, then uses to the default layout. |
|
Provides a title for the object. |
cssClass()
The cssClass()
returns a CSS class for a particular object instance.
The Web UI (Wicket viewer) wraps the object’s representation in a containing <div>
with the class added.
This is done both for rendering the object either in a table or when rendering the object on its own page.
In conjunction with custom CSS (usually CSS in the static/css/application.css
), we can therefore provide custom styling of an object instance wherever it is rendered.
For example, in a todo app we can use this technique to add a strikethrough for completed todo items. This is shown on the home page:
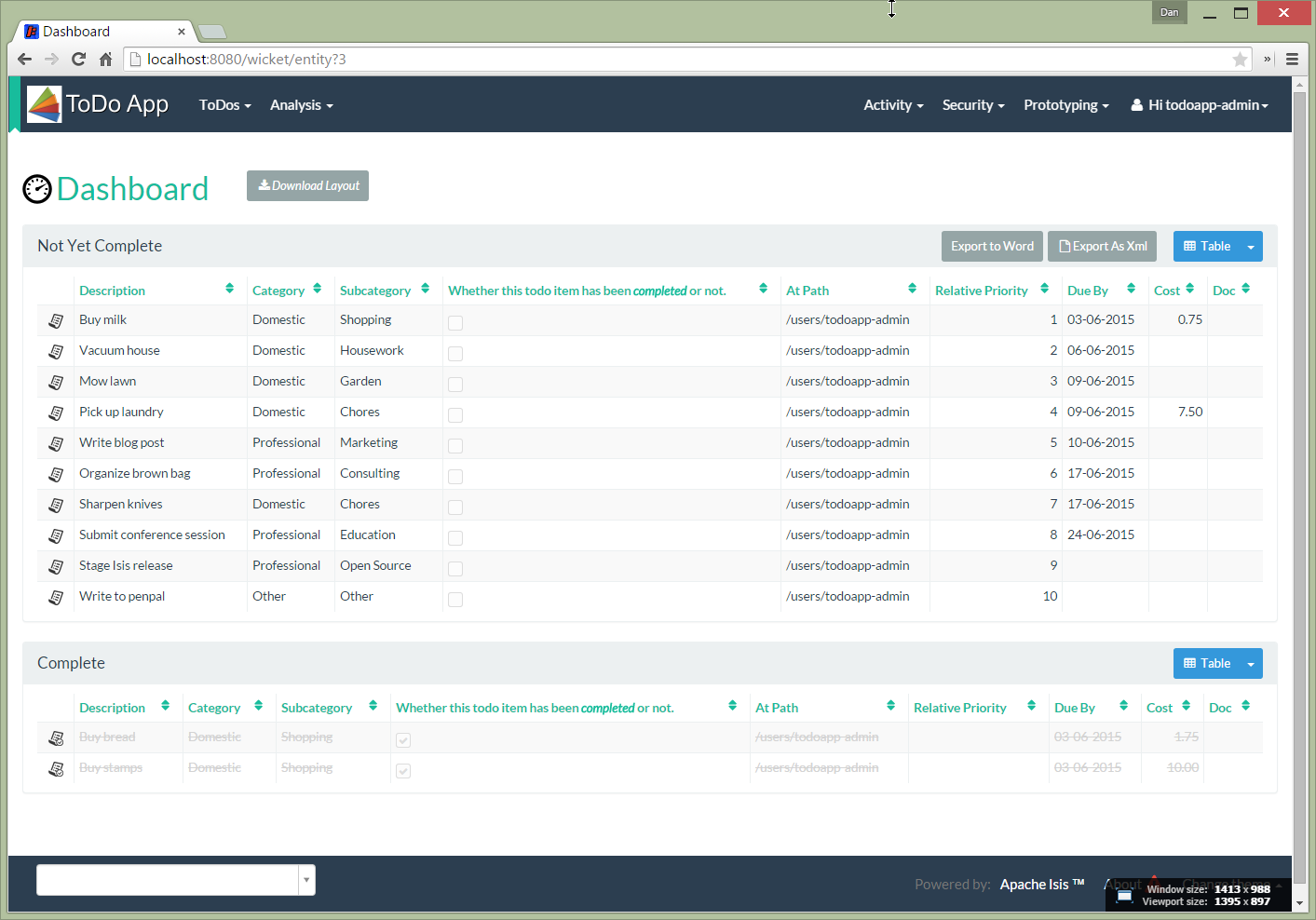
The code to accomplish this is straightforward:
public class ToDoItem ... {
public String cssClass() {
return !isComplete() ? "todo" : "done";
}
...
}
In the application.css, the following styles were then added:
tr.todo {
}
tr.done {
text-decoration: line-through;
color: #d3d3d3;
}
iconName()
Every object is represented by an icon; this is based on the domain object’s simple name.
The Web UI (Wicket viewer) searches for the image in the same package as the .class
file for the domain object or in the images
package.
It will find any matching name and one of the following suffixes .png
, .gif
, .jpeg
, .jpg
or .svg
.
If none is found, then Default.png
will be used as fallback.
The iconName()
allows the icon that to be used to change for individual object instances.
These are usually quite subtle, for example to reflect the particular status of an object.
The value returned by the iconName()
method is added as a suffix to the base icon name.
For example, in a todo app we can use this technique to add an overlay for todo items that have been completed:
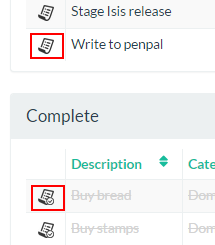
The screenshot below shows the location of these .png
icon files:
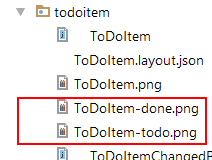
The code to accomplish this is straightforward:
public class ToDoItem ... {
public String iconName() {
return !isComplete() ? "todo" : "done";
}
...
}
layout()
Every object has a layout . This may be specified using annotations such as ActionLayout, PropertyLayout, CollectionLayout, @DomainObjectLayout and the like, but it much more commonly specified using an XML layout file.
The layout
method allows the domain object to specify an alternate layout to its usual layout.
For example:
public class Customer {
@Getter @Setter
@PropertyLayout(hidden=ALWAYS)
private String layout;
public String layout() { (1)
return layout;
}
@ActionLayout(
redirect=EVEN_IF_SAME (2)
)
public Customer switchToEditMode() {
setLayout("edit");
return this;
}
}
1 | specifies the alternate layout to use, eg Customer-edit.layout.xml . |
2 | even though this action returns the same target object, still re-render the page. |
If switchToEditMode()
action is invoked, then the UI will attempt to render the customer using a Customer.layout.edit.xml
layout file (instead of the default Customer.layout.xml
).
title()
Every object is represented by a title. This appears both as a main header for the object when viewed as well as being used as a hyperlink within properties and collections. It therefore must contain enough information for the end-user to distinguish the object from any others.
This is most commonly done by including some unique key within the title, for example a customer’s SSN, or an order number, and so forth. However note that Apache Causeway itself does not require the title to be unique; it is merely recommended in most cases.
An object’s title can be constructed in various ways, but the most flexible is to use the title()
method.
The signature of this method is usually:
public String title() {
// ...
}
Note that Apache Causeway' i18n support extends this so that titles can also be internationalized.
For example, in a todoapp we can use this technique to change the title of items that have been completed:
public String title() {
if (isComplete()) { (1)
return getDescription() + "- Completed!");
} else {
final LocalDate dueBy = getDueBy();
if (dueBy != null) { (2)
return getDescription() + " due by " + dueBy);
} else {
return getDescription();
}
}
}
1 | imperative conditional logic |
2 | imperative conditional logic |
As the example above shows, the implementation can be as complex as you like.
In many cases, though, you may be able to use the @Title annotation.